react-component
Generate a fully functioning React function component.
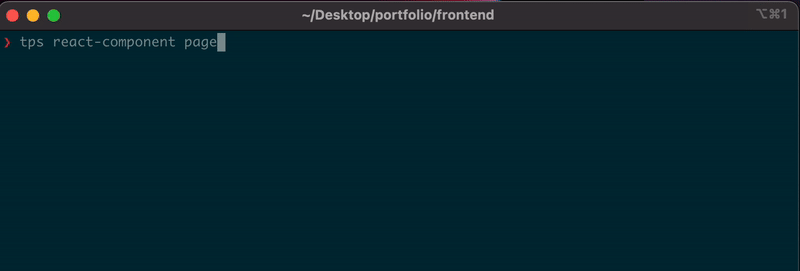
Usage
If you're using our template and have ideas on how we can make it even better, we'd love to hear from you. Your feedback helps us prioritize improvements and build a tool that truly meets your needs.
Feel free to submit feature requests, suggestions, or any thoughts you have using the form below. We hope to hear from you soon!
Submit feature requesttps react-component <component-name>
# or in a directory
tps react-component path/to/dir/<component-name>
| - <component-name>
| - index.js (optional)
| - <component-name>.jsx
| - <component-name>.css (optional)
| - <component-name>.test.js (optional)
| - <component-name>.stories.jsx (optional)
Example
- Default
- In directory
tps react-component Nav
Will produces something like the following:
| - Nav
| - index.js
| - Nav.jsx
| - Nav.css
tps react-component src/components/Nav
Will produces something like the following:
| - src/
| - components/
| - Nav
| - index.js
| - Nav.jsx
| - Nav.css
Check out our examples section to see detailed instructions on how to use this template.
Installation
This templates is a part of Templates library. If you've already installed Templates, you'll have instant access to this template, and you can disregard this command.
npm i -g templates-mo
Options
name | description | option | alias | default | hidden |
---|---|---|---|---|---|
export | Type of export that will be used in your component (default, named) | --export | default | true | |
inlineDefaultExport | Places the default export on the same line as the component, if export is default. | --inlineDefaultExport | false | true | |
functionStyle | Type of function that will be used in your component (function, arrow) | --functionStyle | function | true | |
typescript | Generate the component in TypeScript | --typescript | -t | false | false |
extension | Extension that will be used for the component file | --extension | -e, --ext, --extention | jsx | false |
css | Generate a css file for the component | --css | -c | true | false |
cssExtension | Extension that will be used for the css file | --cssExtension | --cssType, --cssExt | css | false |
test | Generate a test file for the component | --test | false | false | |
reactTestingLibrary | Use react testing library in the test file | --reactTestingLibrary | true | true | |
jestDomImport | Add a @testing-library/jest-dom import statement to the test file | --jestDomImport | false | true | |
testExtension | Extension that will be used for the test file | --testExtension | --testType, --testExt | test.jsx | false |
index | Generate a index file for the component | --index | -i | true | false |
indexExtension | Extension that will be used for the index file | --indexExtension | --indexExt | js | true |
indexExportPattern | Type of export pattern that will be used for the index file (shorthand, explicit) | --indexExportPattern | -i | shorthand | true |
component | Element or component that will be returned in the component file | --component | div | true | |
storybook | Generate a storybook file for the component | --storybook | -s, --story | false | true |
formatter | Type of formatter you would like to use to format the component (none, prettier, biome) | --formatter | --format | none | true |
linter | Type of linter you would like to use to format the component (none, eslint, biome) | --linter | --lint | none | true |
Copy
If you like this template, but want to modify a few things use the copy command. It allows you to duplicate the template into your project and tailor it to your needs.
# if your not initialized run
tps init
# copy template
tps copy react-component
Examples
How to use
Lets say you have a basic react folder structure like so:
| - <cwd>/
| - src/
| - components/
| - ...
| - pages/
| - ...
| - index.jsx
| - app.jsx
| - package.json
| - package-lock.json
If you wanted to generate a new nav component in the components folder, you could use the following command:
tps react-component src/components/Nav
The name you pass in will be used as the name of your folder and component files. If your repository uses param-case, camelCase, or snake_case file naming patterns, you can also use those naming conventions.
# camel case
tps react-component src/components/navItem
# or param case
tps react-component src/components/nav-item
# or snake case
tps react-component src/components/nav_item
Your component name will be the name you passed in converted into Pascalcase.
Once the command is run, you will be prompted some questions:
? Would you like to use typescript? yes
? What type of extension do you want for your component? jsx
? Would you like to include a css file? yes
? What type of css extension would you like? css
? Would you like to include unit tests? no
? Would you like to include a index file? no
? Would you like to include a storybook file? no
Depending on your answers, you'll end up with something similar to the following:
| - <cwd>/
| - src/
| - components/
| - Nav/
| - index.js
| - Nav.jsx
| - Nav.css
| - ...
If you used another naming format, such as
param-case, and created a component
called nav-item
:
tps react-component src/components/nav-item
Depending on your answers, you'll end up with something similar to the following:
| - <cwd>/
| - src/
| - components/
| - nav-item/
| - index.js
| - nav-item.jsx
| - nav-item.css
| - ...
By default, we generate a component with a default export and function declaration, so you will end up with something like the following:
import React, { useEffect, useState } from 'react';
import './Nav.css';
function Nav({}) {
return <div>Nav component</div>;
}
export default Nav;
How to use options
The React component template provides a wide range of options, which are listed in the options table. Most options will be prompted to you when you create a new instance. However, you can also use options on the command line or in your config file.
When using a option on the command line or in a config file, it will no longer be prompted.
example:
If you want to use a js
extension for the component instead of the default
jsx
, you can do one of the following:
- cli
- .tps/.tpsrc
You can pass in the following flags when your generating your new instance:
tps react-component Nav --extension=js
# or
tps react-component Nav --extension js
Boolean options can be used by providing the option name for true
, or by
prefixing the option name with --no-
to specify false
.
# true
--typescript
# false
--no-typescript
Depending on the rest of your answers, it will generate something similar to the following:
| - Nav
| - index.js
| - Nav.js
| - Nav.css
You can add the following to your .tps/.tpsrc
file to always use a js
extension for your component when generating a new instance in your repo.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"extension": "js"
}
}
}
Depending on the rest of your answers, it will generate something similar to the following:
| - Nav
| - index.js
| - Nav.js
| - Nav.css
Hidden options
Hidden options dont get prompted by default. However, you can always answer
hidden options on the command line or in your config file.
Additionally, you can use the hidden
option
to have all hidden
options prompted.
No new folder
By default, templates creates a new folder for you. If you don't want a new
folder for your react component, you can use the
newFolder
and
index
options. These options both default to
true
.
The newFolder
option tells templates whether
or not to place everything into a new folder.
The index
option determines whether a index
file should be created.
Lets say you have a basic react folder structure like so:
| - <cwd>/
| - src/
| - components/
| - Nav.jsx
| - Nav.css
| - pages/
| - ...
| - index.jsx
| - app.jsx
| - package.json
| - package-lock.json
If you wanted to generate a new component called footer
in the components
folder without a new folder, you can do one of the following:
- cli
- .tps/.tpsrc
You can pass in the following flags when your generating your new instance
tps react-component footer --no-newFolder --no-index
You can add the following to your .tps/.tpsrc
file to always avoid creating a
new folder when generating a React component in your repository directory and
all its subdirectories.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"index": false
},
"opts": {
"newFolder": false
}
}
}
Now when generating a new instance
tps react-component footer
Depending on your answers, it would produce something to the following:
| - <cwd>/
| - src/
| - components/
| - nav.jsx
| - nav.css
| - footer.jsx
| - footer.css
| - pages/
| - ...
| - index.jsx
| - app.jsx
| - package.json
| - package-lock.json
How to use different export styles
By default, this template uses a default export when exporting your new
component. If your repo uses a different export pattern, you can configure it
with the export
option. Currently, only default
and named
exports are
supported. You can specify this either on the command line or in your
config file file.
To use a named export for your component, you can do one of the following:
- cli
- .tps/.tpsrc
You can pass in the following flag when your generating your new instance:
tps react-component Nav --export named
You can add the following to your .tps/.tpsrc
file to always use named exports
when generating a new component.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"export": "named"
}
}
}
tps react-component Nav
Now, when your component is rendered, it will use a named export, similar to the following:
import React, { useEffect, useState } from 'react';
import './Nav.css';
export function Nav({}) {
return <div>Nav component</div>;
}
Inline default exports
This template also supports inline default export statements. You can use a
inline default export by using the inlineDefaultExport
option. You can specify
this either on the command line or in your config file file.
- cli
- .tps/.tpsrc
You can pass in the following flag when your generating your new instance:
tps react-component Nav --inlineDefaultExport
You can add the following to your .tps/.tpsrc
file to always use inline
default exports when generating a new component.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"inlineDefaultExport": true
}
}
}
tps react-component Nav
Now, when your component is rendered, it will use a inline default export, similar to the following:
import React, { useEffect, useState } from 'react';
import './App.css';
export default function App({}) {
return <div>App component</div>;
}
How to use with typescript
The typescript
option controls whether the component will be rendered using
typescript or not.
When your component uses typescript it will add a typescript interface for the
component props and changes the default value for the extension
option to
tsx
.
When generating a new instance of this template, you will be asked if you want
to use Typescript. However, if you know your going to use typescript and you
dont want to be prompted every time, set the typescript
option on the command
line or in your config file.
Only the default value for extension
will be changed and this prompt will
still be asked when generating new instances. If dont want this prompt to be
prompted, set the extension
option in your config file or on
the command line.
- cli
- .tps/.tpsrc
You can pass in the following flags when your generating your new instance and you will no longer be prompted this question.
tps react-component Nav --typescript
# or
tps react-component Nav -t
You can add the following to your .tps/.tpsrc
file to always use typescript
when generating a react component in your repo.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"typescript": true
}
}
}
Now when generating a new instance you no longer will be prompted for these two questions or have to supply the two flags to the cli command.
tps react-component Nav
Depending on your answers, it would produce something to the following:
| - Nav
| - index.ts
| - Nav.tsx
| - Nav.css
Your component file will end up with something like the following:
import React, { useEffect, useState } from 'react';
import './Nav.css';
interface NavProps {
// props
}
function Nav({}: NavProps) {
return <div>Nav component</div>;
}
export default Nav;
How to use different function styles
By default, this template uses a function declaration for your new component. If
your repo uses a different function pattern, you can configure it with the
functionStyle
option. Currently, only arrow
functions and function
declarations are supported. You can specify this either on the command line or
in your config file file.
To use an arrow function for your component, you can do one of the following:
- cli
- .tps/.tpsrc
You can pass in the following flag when your generating your new instance:
tps react-component Nav --functionStyle arrow
You can add the following to your .tps/.tpsrc
file to always use arrow
functions when generating a new component.
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"functionStyle": "arrow"
}
}
}
tps react-component Nav
Now, when your component is rendered, it will use an arrow function, similar to the following:
import React, { useEffect, useState } from 'react';
import './Nav.css';
const Nav = ({}) => {
return <div>Nav component</div>;
};
export default Nav;
Formatting (beta)
By default, this template does not automatically run any formatters (e.g.,
Prettier). However, you can enable automatic formatting
using the formatter
option. Currently, only prettier
, biome
, and none
are supported. You can specify this either on the command line or in your
config file file.
To enable Prettier formatting, follow one of these methods:
- cli
- .tps/.tpsrc
You can pass in the following flag when your generating your new instance:
tps react-component Nav --formatter prettier
You can add the following to your .tps/.tpsrc
file to always run prettier
formatting when generating new instances:
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"formatter": "prettier"
}
}
}
tps react-component Nav
Now, after your new component is created, we will run prettier against the newly created files.
When not using a new folder, please note that we will run the formatter against your current working directory. We are working to improve this experience.
Linting (beta)
By default, this template does not automatically run any linters (e.g.,
eslint). However, you can enable lint fixing by using the
linter
option. Currently, only eslint
, biome
, and none
are supported.
You can specify this either on the command line or in your
config file file.
To enable eslint fixing, follow one of these methods:
- cli
- .tps/.tpsrc
You can pass in the following flag when your generating your new instance:
tps react-component Nav --linter eslint
You can add the following to your .tps/.tpsrc
file to always run eslint
fixing:
If you havent already initialize your repo:
tps init
{
"react-component": {
"answers": {
"linter": "eslint"
}
}
}
tps react-component Nav
Now, after your new component is created, we will run eslint fix against the newly created files.
When not using a new folder, please note that we will run the linter against your current working directory. We are working to improve this experience.
Use all default answers
To bypass all prompts and use default answers, simply include the --default
flag when generating a new instance.
tps react-component --default
You can also combined this with other flags and/or in conjunction with your
.tps/.tpsrc
- cli
- .tps/.tpsrc
Use typescript and use defaults for the rest of the questions. This will no longer prompt you any questions
tps react-component Nav --typescript --default
If you have typescript set in your tpsrc file.
{
"react-component": {
"answers": {
"typescript": true
}
}
}
Now when using defaults it will use the typescript, defaults for the rest of the questions, and will no longer prompt any questions.
tps react-component Nav --default